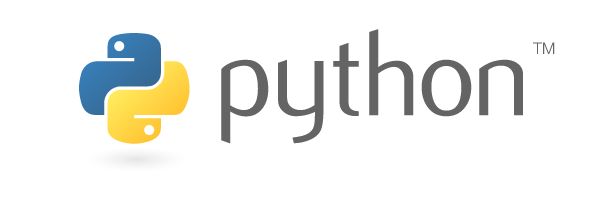
本文主要用于记录LeetCode中遇到的比较好的一些python写法以及招聘笔试面试中遇到的问题。
self参数
在python的类的方法定义中,请描述’self’参数的作用?
self参数在类定义中,指类本身,可以用来传递外部参数。
math库
log函数
描述:
log()
返回 x 的自然对数。
语法:
1 | import math |
参数:
- x – 数值表达式。
- base – 可选,底数,默认为 e。
返回值:
返回 x 的自然对数,x>0。
实例:
1 | import math |
sum函数
描述:
sum() 方法对系列进行求和计算。
语法:
sum(iterable[, start])
参数:
- iterable – 可迭代对象,如:列表、元组、集合。
- start – 指定相加的参数,如果没有设置这个值,默认为0。
返回值:
返回计算结果。
实例:
1 | sum([0,1,2]) |
常见用法:
将程序变得更pythic,以171.Excel表列序号
为例:
- 常规
1 | res = 0 |
- sum()
1 | return sum((26 ** (len(s) - index - 1)) * (ord(s[index]) - ord('A') + 1) for index in range(len(s))) |
把每次迭代的结果以sum的方式一次性取和。
ord函数
描述:
它以一个字符(长度为1的字符串)作为参数,返回对应的 ASCII 数值,或者 Unicode 数值,如果所给的 Unicode 字符超出了你的 Python 定义范围,则会引发一个 TypeError 的异常。
语法:
1 | ord(c) |
参数:
c – 字符
返回值:
返回值是对应的十进制整数。
实例:
1 | ord('a') |
排序
数组倒序
1 | a = [1, 2, 3, 4, 5] |
sort函数
sort()是Python列表(仅适用于列表)的一个内置的排序方法,list.sort()方法排序时直接修改原列表,返回None;
语法:
1 | list.sort(*, key=None, reverse=None) |
参数:
- key
带一个参数的函数,返回一个值用来排序,默认为 None - reverse
表示排序结果是否反转,默认是升序
实例:
1 | a=['1',1,'a',3,7,'n'] |
sorted函数
sorted()是迭代器(不仅针对列表)的排序方法,可以保留原列表,返回新列表。
语法:
1 | sorted(iterable[, key][, reverse]) |
参数:
- key
带一个参数的函数,返回一个值用来排序,默认为 None - reverse
表示排序结果是否反转
实例:
1 | a = (1,2,4,2,3) |
set
数据类型set是一个无序不重复元素集。
基本功能包括关系测试和消除重复元素。
集合对象支持联合、交、差和对称差集等数学运算。
支持:
- x in set
- len(set)
- for x in set
不支持:
- indexing
- slicing
- 其它类序列(sequence-like)的操作
元素集合
1 | x = set('spam') |
交集
1 | x = set('spam') |
交集
1 | x = set('spam') |
差集
1 | x = set('spam') |
对称差集
1 | x = set('spam') |
去除重复元素
1 | x = set('spam') |
添加元素
1 | # 添加一项 |
删除元素
1 | #删除一项 |
set 的长度
1 | len(x) |
关系测试
1 | s in x # 测试 s 是否是 x 的成员 |
zip函数
描述:
从参数中的多个迭代器取元素组合成一个新的迭代器。
语法:
1 | zip(iterable,[iterable...]) |
注意:
当有多个参数时,zip(a,b)
中a和b的维数相同,若不相同时,取最小的维数进行组合。
参数:
一个或多个迭代器,例如,元组、列表、字典等。
返回值:
返回一个zip对象,其内部元素为元组;
可以转化为元组、列表、字典
实例:
- 单个参数
1 | list1 = [1, 2, 3, 4] |
- 多个参数
1 | m = [[1,2,3], [4,5,6], [7,8,9]] |
*zip(*iterables)
描述:
*zip()函数是zip()函数的逆过程,将zip对象变成原先组合前的数据。
实例:
1 | m = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] |
zip(*)
1 | p = [[1,2,3],[4,5,6]] |
sys模块
sys.stdin
标准输入:
stdin
获得输入(用input()输入的通过它获得)
- readline()
每次读取一整行,包括 “\n” 字符。 - readlines()
读取所有行并返回列表,包括 “\n” 字符。
sys.stdout
stdout
用于输出print()和expression语句以及input()的提示。
print()
的本质就是sys.stdout.write(object + ‘\n’)
删除指定字符
strip函数
移除字符串头尾指定的字符(默认为空格或换行符)或字符序列。
注意:该方法只能删除开头或是结尾的字符,不能删除中间部分的字符。
语法:
1 | str.strip([chars]) |
参数:
chars:移除字符串头尾指定的字符序列。
返回值:
返回移除字符串头尾指定的字符生成的新字符串。
实例:
1 | # 去除首尾字符 0 |
replace函数
实则为替代函数,但也非常方便实施删除指定元素。
语法:
1 | str.replace(old, new[, max]) |
参数:
- old – 将被替换的子字符串。
- new – 新字符串,用于替换old子字符串。
- max – 可选字符串, 替换不超过 max 次
返回值:
返回字符串中的 old(旧字符串) 替换成 new(新字符串)后生成的新字符串,如果指定第三个参数max,则替换不超过 max 次。
实例:
1 | #展示替换功能 |
functools库
map函数
描述:
map()
会根据提供的函数对指定序列做映射。
语法:
1 | map(function, iterable, ...) |
参数:
- function – 函数,有两个参数
- iterable – 一个或多个序列
返回值:
Python 3.x 返回迭代器。
实例:
1 | def square(x) : # 定义计算平方数的函数 |
总结:
map(function, iterable, …)
相当于[f(x) for x in list]
reduce函数
对参数序列中的元素进行累积。
将序列中的第一个元素与第二个元素进行操作,得到的结果再与第三个元素进行运算。
语法:
1 | reduce(function, iterable[, initializer]) |
参数:
- function – 函数,有两个参数
- iterable – 可迭代对象
- initializer – 可选,初始参数
返回值:
返回函数计算结果。
实例:
1 | #实现数组内所有元素相乘 |